102. Binary Tree Level Order Traversal
Problem
Given the root
of a binary tree, return the level order traversal of its nodes' values. (i.e., from left to right, level by level).
Example 1:
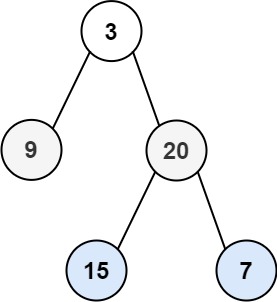
Input: root = [3,9,20,null,null,15,7] Output: [[3],[9,20],[15,7]]
Example 2:
Input: root = [1] Output: [[1]]
Example 3:
Input: root = [] Output: []
Constraints:
- The number of nodes in the tree is in the range
[0, 2000]
. -1000 <= Node.val <= 1000
Solution
With recursion:
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, val=0, left=None, right=None):
# self.val = val
# self.left = left
# self.right = right
class Solution:
def levelOrder(self, root: Optional[TreeNode]) -> List[List[int]]:
levels = []
if not root:
return levels
def helper(node, level):
# start the current level
if len(levels) == level:
levels.append([])
# append the current node value
levels[level].append(node.val)
# process child nodes for the next level
if node.left:
helper(node.left, level + 1)
if node.right:
helper(node.right, level + 1)
helper(root, 0)
return levels
This is a bit confusing and I don't like it and I didn't come up with it. I'd prefer a BFS method with a queue.
BFS
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, val=0, left=None, right=None):
# self.val = val
# self.left = left
# self.right = right
class Solution:
def levelOrder(self, root: Optional[TreeNode]) -> List[List[int]]:
res = []
# queue
q = collections.deque()
q.append(root)
while q:
# while queue is non-empt
qLen = len(q)
# loop through all values in the queue
# makes sure we iterate one level at a time
level = []
# for all nodes in that level
for i in range(qLen):
node = q.popleft()
# FIFO by popping left
if node:
level.append(node.val)
# technically these could be null, hence the if node above
q.append(node.left)
q.append(node.right)
if level:
res.append(level)
return res