110. Balanced Binary Tree
Problem
Given a binary tree, determine if it is
height-balanced.
Example 1:
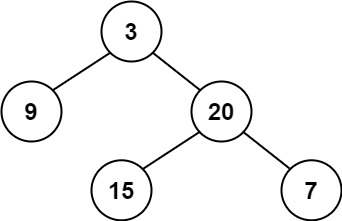
Input: root = [3,9,20,null,null,15,7] Output: true
Example 2:
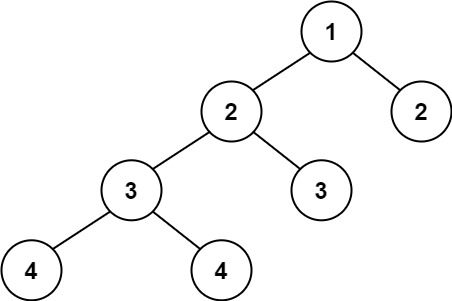
Input: root = [1,2,2,3,3,null,null,4,4] Output: false
Example 3:
Input: root = [] Output: true
Solution
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, val=0, left=None, right=None):
# self.val = val
# self.left = left
# self.right = right
class Solution:
def isBalanced(self, root: Optional[TreeNode]) -> bool:
def check(root):
if root is None:
return 0
# exhaust both left and right trees
left = check(root.left)
right = check(root.right)
# check if our trees are unbalanced
# we use abs because one side might be more than the other
# resulting in a negative number
if left == -1 or right == -1 or abs(left - right) > 1:
return -1
# Increment by one since we have counted a node
return 1 + max(left, right)
return check(root) != -1