235. Lowest Common Ancestor of a Binary Search Tree
Problem
Given a binary search tree (BST), find the lowest common ancestor (LCA) node of two given nodes in the BST.
According to the definition of LCA on Wikipedia: “The lowest common ancestor is defined between two nodes p
and q
as the lowest node in T
that has both p
and q
as descendants (where we allow a node to be a descendant of itself).”
Example 1:
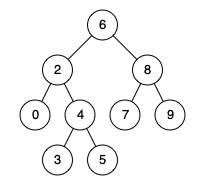
Input: root = [6,2,8,0,4,7,9,null,null,3,5], p = 2, q = 8 Output: 6 Explanation: The LCA of nodes 2 and 8 is 6.
Example 2:
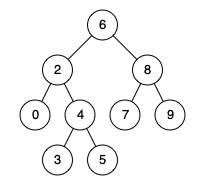
Input: root = [6,2,8,0,4,7,9,null,null,3,5], p = 2, q = 4 Output: 2 Explanation: The LCA of nodes 2 and 4 is 2, since a node can be a descendant of itself according to the LCA definition.
Example 3:
Input: root = [2,1], p = 2, q = 1 Output: 2
Solution
2 important things to note:
- We start at the root, because an ancestor is always higher so must always start at the root.
- it's a binary search tree.
If we're looking to find p = 2
, it'll be in the left subtree because 2 is less than 6 (binary search tree property).
# Definition for a binary tree node.
# class TreeNode(object):
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution(object):
def lowestCommonAncestor(self, root, p, q):
"""
:type root: TreeNode
:type p: TreeNode
:type q: TreeNode
:rtype: TreeNode
"""
curr = root
# p and q are gurnateed to exist in the tree
# makes it run forever until we find the result
while curr:
# if p and q greater than root, it's right subtree
if p.val > curr.val and q.val > curr.val:
curr = curr.right
elif p.val < curr.val and q.val < curr.val:
# elif its left subtree
curr = curr.left
else:
# we found the split where we have found our result
return curr
# no return stateemnt outside of loop as it's guranteed to exist
# see the constraints
# > p and q will exist in the BST.