733. Flood Fill
Problem
An image is represented by an m x n
integer grid image
where image[i][j]
represents the pixel value of the image.
You are also given three integers sr
, sc
, and color
. You should perform a flood fill on the image starting from the pixel image[sr][sc]
.
To perform a flood fill, consider the starting pixel, plus any pixels connected 4-directionally to the starting pixel of the same color as the starting pixel, plus any pixels connected 4-directionally to those pixels (also with the same color), and so on. Replace the color of all of the aforementioned pixels with color
.
Return the modified image after performing the flood fill.
Example 1:
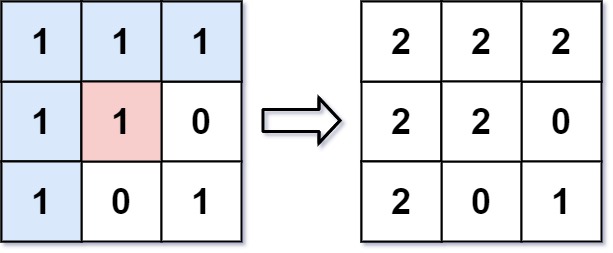
Input: image = [[1,1,1],[1,1,0],[1,0,1]], sr = 1, sc = 1, color = 2 Output: [[2,2,2],[2,2,0],[2,0,1]] Explanation: From the center of the image with position (sr, sc) = (1, 1) (i.e., the red pixel), all pixels connected by a path of the same color as the starting pixel (i.e., the blue pixels) are colored with the new color. Note the bottom corner is not colored 2, because it is not 4-directionally connected to the starting pixel.
Example 2:
Input: image = [[0,0,0],[0,0,0]], sr = 0, sc = 0, color = 0 Output: [[0,0,0],[0,0,0]] Explanation: The starting pixel is already colored 0, so no changes are made to the image.
Constraints:
m == image.length
n == image[i].length
1 <= m, n <= 50
0 <= image[i][j], color < 216
0 <= sr < m
0 <= sc < n
Solution
The solution is rather simplistic (if hard to read in code). We find the source cell and perform a depth first search on it.
We continue the depth first search if:
- The next is within bounds (its up, down, left, or right of our source cell)
- The next cell is the same colour as the source cell was
class Solution(object):
def floodFill(self, image, sr, sc, color):
"""
:type image: List[List[int]]
:type sr: int
:type sc: int
:type color: int
:rtype: List[List[int]]
"""
# The idea is simple. Simply perform a DFS on the source cell. Continue the DFS if:
# Next cell is within bounds.
# Next cell is the same color as source cell.
xlen = len(image)
ylen = len(image[0])
ori = image[sr][sc]
def dfs(x, y):
if 0<=x<xlen and 0<=y<ylen and image[x][y]==ori:
# set source cell to new color
image[x][y] = color
# DFS over all neighbours
dfs(x-1, y)
dfs(x+1, y)
dfs(x, y-1)
dfs(x, y+1)
if ori != color:
dfs(sr, sc)
return image